Sending email in GO using Smtp API
Experience a breakthrough in your development process with TurboSMTP's Email API. Achieve swift integration and instant email dispatch. Our API, compatible with any GO application, streamlines large-scale email sending with easy-to-follow documentation.
Email API for GO Developers
TurboSMTP's Email API simplifies the integration of email functionalities into your GO applications. Featuring a user-friendly API, smooth integration, and detailed documentation, it enables you to efficiently scale your email delivery, from a couple of emails to millions. Below, you'll find straightforward examples demonstrating the integration of our APIs with GO applications.
package main
import (
"bytes"
"fmt"
"io/ioutil"
"net/http"
)
func main() {
// setup.
url := "https://api.turbo-smtp.com/api/v2/mail/send"
consumerkey := "<CONSUMER_KEY>"
consumerSecret := "<CONSUMER_SECRET>"
// Body Data.
data := []byte(`{
"from": "hello@your-company.com",
"to": "Doe.Jhon@gmail.com,contact@global-travel.com",
"subject": "New live training session",
"cc": "cc_user@example.com",
"bcc": "bcc_user@example.com",
"content": "Dear partner,\nWe are delighted to invite you to an exclusive training session on UX Design. This session is designed to provide essential insights and practical strategies to enhance your skills.",
"html_content": "Dear partner,\nWe are delighted to invite you to an exclusive training session on <strong>UX Design</strong>. This session is designed to provide essential insights and practical strategies to enhance your skills."
}`)
// Create POST Resquest.
req, err := http.NewRequest("POST", url, bytes.NewBuffer(data))
if err != nil {
fmt.Println("An Error has occured:", err)
return
}
// Set Request Headers.
req.Header.Set("Content-Type", "application/json")
req.Header.Set("accept", "application/json")
req.Header.Set("consumerKey", consumerkey)
req.Header.Set("consumerSecret", consumerSecret)
// Perform HTTP Request.
client := &http.Client{}
resp, err := client.Do(req)
if err != nil {
fmt.Println("An Error has occured sending the request:", err)
return
}
defer resp.Body.Close()
// Read Server Response
body, err := ioutil.ReadAll(resp.Body)
if err != nil {
fmt.Println("An Error has occured reading the server response:", err)
return
}
// Print Server Response
fmt.Println(string(body))
}
Send Emails Using Your Favorite Coding Language
Our APIs offer developers the flexibility to effortlessly integrate email solutions using their preferred programming languages. Whether you're skilled in Python, cURL, Ruby, or any other popular coding language, our comprehensive API support has you covered.
TurboSMTP Service Highlights
Here are four compelling reasons to choose TurboSMTP's service: unparalleled email delivery, data-driven insights through analytics, robust privacy and security, and dedicated 24/7 support for a worry-free email experience.
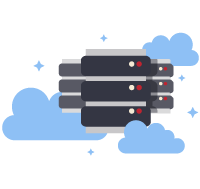
Powerful Infrastructure for High Email Deliverability
Our infrastructure scales for mass email distribution, offering SMTP and API integration, with TurboSMTP ensuring fast, effective communication.
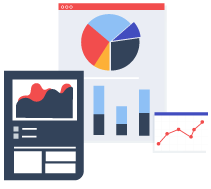
Refine Outreach with Email Metrics & Analytics
TurboSMTP's tools provide insights on email performance, helping optimize campaigns for better engagement, conversions, and ROI through data-driven strategies.
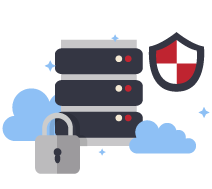
Email Privacy and Security: Fundamental Values
Our email service prioritizes privacy and security, using advanced encryption and strict privacy law adherence to protect your data and communications.
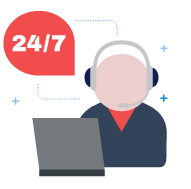
Support at Your Service Whenever You Want
TurboSMTP provides 24/7 collaborative support with a dedicated team, ensuring a smooth, worry-free email experience and reliable assistance at every step.
Get Started with TurboSMTP Email API
Just follow these three simple steps to seamlessly integrate TurboSMTP into your GO application.
Sign up for a free account and get 10,000 free emails per month.
Retrieve your API key from your dashboard.
Follow our API documentation to start your integration.